The Standard Function Library: This library consists of general-purpose,stand-alone functions that are not part of any class. The function library is inherited from C, it is a set of C++ template classes to provides general-purpose empathized classes and functions that implement commonly used algorithms and data structures like vectors, lists, and stacks.
Vectors are array which can be change in size; compared to arrays, vectors consume more memory in exchange for the ability to manage storage and grow dynamically in an efficient way.
Containers : Containers are used to manage collections of objects of a certain kind. We Use <vector> in our syllabus.
Algorithms : Algorithms act on containers. They provide the means by which you will perform initialization, sorting, searching, and transforming of the contents of containers.
Iterators : Iterators are used to step through or move pointer in a container.
- The push_back( ) member function inserts value at the end of the vector, expanding its size as needed.
- The size( ) function displays the size of the vector.
- The function begin( ) returns an iterator to the start of the vector.
- The function end( ) returns an iterator to the end of the vector.
- The function empty() returns whether the vector is empty (i.e. whether its size is 0).
A NORMAL PROGRAM IS HERE TO GET INPUT IN A VECTOR, DISPLAY THAT VECTOR, INPUT VALUE AT PARTICULAR POSITION;
#include"conio.h"
#include"iostream"
#include"vector" //HEADER FILE FOR VECTOR
using namespace std;
int main()
{
vector <int> v; //DECLARING VECTOR
int i,n,l,num;
cout<<"\nENTER TOTAL NUMBER OF ELEMENT : ";
cin>>n;
for(i=0;i<n;i++)
{
cout<<"\nEnter Value : ";
cin>>num;
v.push_back(num); //USING push_back FUNCTION TO PUT NO. IN VECTOR
}
cout<<"\n_______VALUES ARE________"<<endl;
for(i=0;i<n;i++)
{
cout<<"\nValue at "<<i+1<<" is "<<v[i]<<endl;
}
cout<<"\nEnter Value To Insert At Last Position : ";
cin>>num;
v.push_back(num);
for(i=0;i<v.size();i++)
{
cout<<"\nValue at "<<i+1<<" is "<<v[i]<<endl;
}
cout<<endl;
vector <int> :: iterator ie=v.begin(); //DEFINING iterator TO STARTING OF VECTOR USING <vector>.begin() function
cout<<"\nEnter Steps You Want To Move Down : ";
cin>>l;
ie=ie+l; //SETTING VALUE TO STEP THROUGH
cout<<"\nEnter Value to Insert at "<<l<<" Steps Down : ";
cin>>num;
v.insert(ie,num); INSERTING VALUE AT DEFINED POSITION USING <vector>.insert();
cout<<endl;
for(i=0;i<v.size();i++)
{
cout<<"\nValue at "<<i+1<<" is "<<v[i]<<endl;
}
cout<<"\n\nPROGRAMMING @ C#ODE STUDIO ";
getch();
}
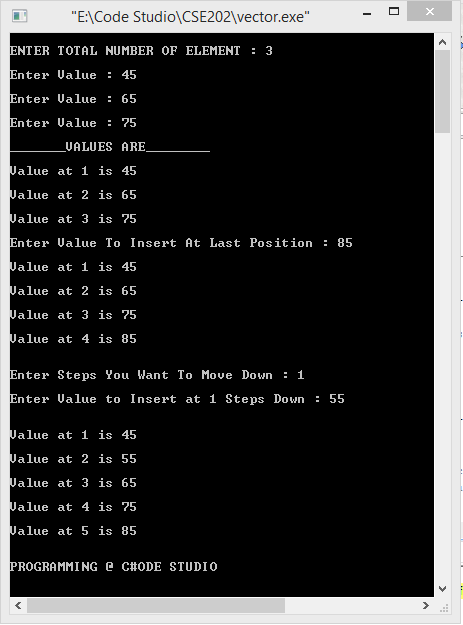